问题描述
给定一个二叉树,要求输出所有二叉树的路径(从根节点到叶子节点的路径),题目链接:点我
样例输入输出
输入:root = [1,2,3,null,5]
输出:[“1->2->5”,”1->3”]
解释:输入的二叉树如下
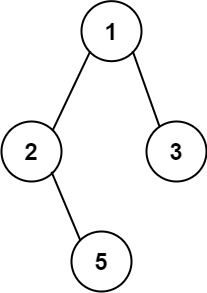
输入:root = [1]
输出:[1]
问题解法
使用先序遍历方式,将每个节点添加到路径上。代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43
|
class Solution { public List<String> binaryTreePaths(TreeNode root) { if (root.left == null && root.right == null) { List<String> result = new ArrayList<>(); result.add(String.valueOf(root.val)); return result; }
List<String> result = new ArrayList<>(); if (root.left != null) { List<String> left = binaryTreePaths(root.left); merge(result, left, String.valueOf(root.val)); }
if (root.right != null) { List<String> right = binaryTreePaths(root.right); merge(result, right, String.valueOf(root.val)); }
return result; }
private void merge(List<String> result, List<String> origin, String value) { for (String item : origin) { result.add(value + "->" + item); } } }
|