问题描述
给定两个链表,要求判断两个链表是否相交,如果相交,则返回相交的节点,否则返回 null
。题目链接:点我
样例输入输出
输入:intersectVal = 8, listA = [4,1,8,4,5], listB = [5,6,1,8,4,5], skipA = 2, skipB = 3
输出:8
解释:两个链表如下所示
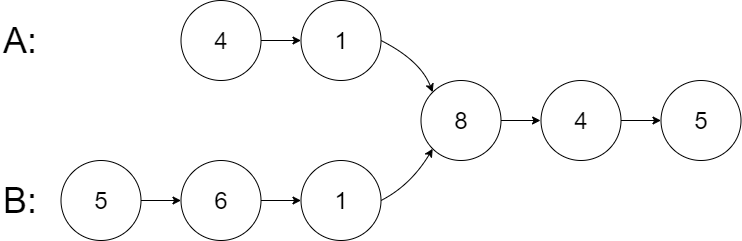
输入:intersectVal = 2, listA = [1,9,1,2,4], listB = [3,2,4], skipA = 3, skipB = 1
输出:2
解释:两个链表如下所示
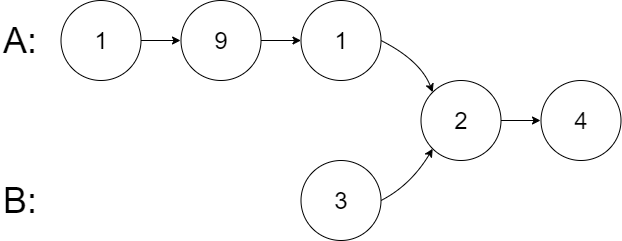
问题解法
先分别计算两个链表的长度,然后将链表长度对齐,从对齐节点开始依次向后遍历链表直到两个指针指向的节点相同(同个节点或者都是null
),代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46
|
public class Solution { public ListNode getIntersectionNode(ListNode headA, ListNode headB) { int lengthA = calcLength(headA); int lengthB = calcLength(headB); ListNode pA = headA; ListNode pB = headB; while (lengthA > lengthB) { pA = pA.next; lengthA--; } while (lengthB > lengthA) { pB = pB.next; lengthB--; } while (pA != pB) { pA = pA.next; pB = pB.next; } return pA; } private int calcLength(ListNode head) { int length = 0; ListNode p = head; while (p != null) { length++; p = p.next; } return length; } }
|