问题描述
给定一个链表,要求判断链表是否存在环。题目链接:**点我**
样例输入输出
输入: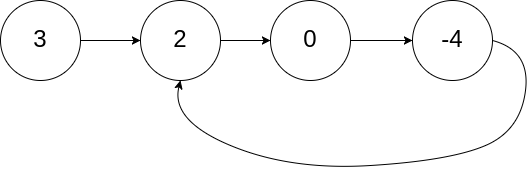
输出:true
输入:1
输出:false
问题解法
此题是判断链表是否存在循环的经典解法,使用快慢指针进行判断,如果两指针相遇,就说明存在循环,否则不存在循环。代码如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31
|
public class Solution { public boolean hasCycle(ListNode head) { if (head == null) { return false; } ListNode left = head; ListNode right = head.next; while (right != null && right.next != null) { right = right.next.next; left = left.next; if (left == right) { return true; } } return false; } }
|